S3 Authentication
Learn about authenticating with Supabase Storage S3.
You have two options to authenticate with Supabase Storage S3:
- Using the generated S3 access keys from your project settings (Intended exclusively for server-side use)
- Using a Session Token, which will allow you to authenticate with a user JWT token and provide limited access via Row Level Security (RLS).
S3 access keys
Keep these credentials secure
S3 access keys provide full access to all S3 operations across all buckets and bypass RLS policies. These are meant to be used only on the server.
To authenticate with S3, generate a pair of credentials (Access Key ID and Secret Access Key), copy the endpoint and region from the project settings page.
This is all the information you need to connect to Supabase Storage using any S3-compatible service.
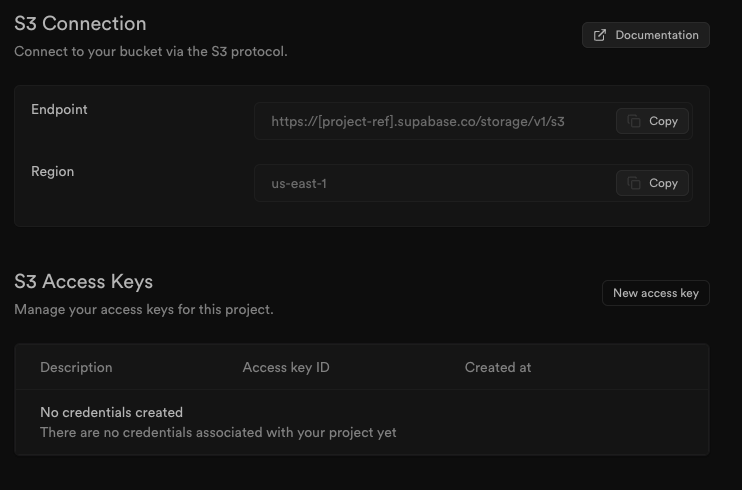
_11import { S3Client } from '@aws-sdk/client-s3';_11_11const client = new S3Client({_11 forcePathStyle: true,_11 region: 'project_region',_11 endpoint: 'https://project_ref.supabase.co/storage/v1/s3',_11 credentials: {_11 accessKeyId: 'your_access_key_id',_11 accessSecretKey: 'your_secret_access_key',_11 }_11})
Session token
You can authenticate to Supabase S3 with a user JWT token to provide limited access via RLS to all S3 operations. This is useful when you want initialize the S3 client on the server scoped to a specific user, or use the S3 client directly from the client side.
All S3 operations performed with the Session Token are scoped to the authenticated user. RLS policies on the Storage Schema are respected.
To authenticate with S3 using a Session Token, use the following credentials:
- access_key_id:
project_ref
- access_secret_key:
anonKey
- session_token:
valid jwt token
For example, using the aws-sdk
library:
_16import { S3Client } from '@aws-sdk/client-s3'_16_16const {_16 data: { session },_16} = await supabase.auth.getSession()_16_16const client = new S3Client({_16 forcePathStyle: true,_16 region: 'project_region',_16 endpoint: 'https://project_ref.supabase.co/storage/v1/s3',_16 credentials: {_16 accessKeyId: 'project_ref',_16 accessSecretKey: 'anonKey',_16 sessionToken: session.access_token,_16 },_16})