Back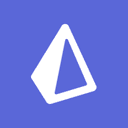
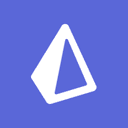
Prisma
Overview
Prisma is an open source next-generation ORM. It consists of the following parts:
- Prisma Client: Auto-generated and type-safe query builder for Node.js and TypeScript.
- Prisma Migrate: Migration system.
- Prisma Studio: GUI to view and edit data in your database.
Documentation
To use Prisma with Supabase, follow our Prisma quickstart guide.
Details
Third-party integrations and docs are managed by Supabase partners.