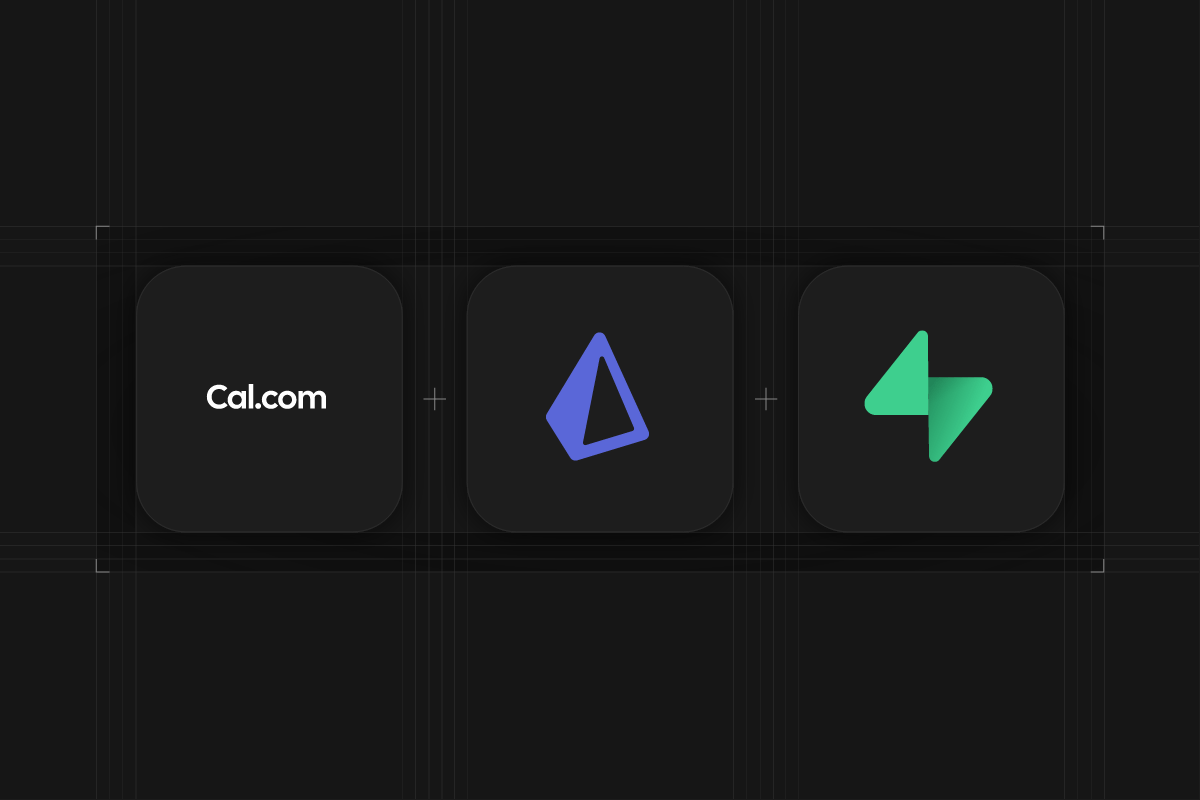
Contrary to popular belief, due to the biggest online beef marketing campaign in modern history, Cal.com and Supabase are actually supa good friends, united by a common mission to build open source software.
So when the Cal.com team reached out about collaborating on their new platform starter kit, we were excited to work together. Finally we could collaborate on a Product Hunt launch instead of competing against each other.
What's the stack?
Initially the application was built to be run on SQLite. However, once requirements grew to include file storage, the Cal.com team remembered their Supabase frenemies and luckily, thanks to Prisma and Supabase, switching things over to Postgres three days before launch was a breeze.
Prisma configuration for usage with Postgres on Supabase
When working with Prisma, your application will connect directly to your Postgres databases hosted on Supabase. To handle connection management efficiently, especially when working with serverless applications like Next.js, Supabase provides a connection pooler called Supavisor to make sure your database runs efficiently with increasing traffic.
The configuration is specified in the schema.prisma
file where you provide the following connection strings:
This loads the relevant Supabase connections strings from your .env
file
You can find the values in the project connect page of your Supabase Dashboard.
For more details on using Prisma with Supabase, read the official docs.
Multischema support in Prisma
In Supabase the public
schema is exposed via the autogenerated PostgREST API, which allows you to connect with your database from any environment that speaks HTTPS using the Supabase client libraries like supabase-js for example.
Since Prisma connects directly to your database, it's advisable to put your data on a separate schema that is not exposed via the API.
We can do this by enabling multischema
support in the schema.prisma
file:
React Dropzone and Supabase Storage for profile image uploads
Supabase Storage is an S3 compatible cloud-based object store that allows you to store files securely. It is conveniently integrated with Supabase Auth allowing you to easily limit access for uploads and downloads.
Cal.com's Platforms Starter Kit runs their authentication on Next.js' Auth.js. Luckily though, Supabase Storage is supa flexible, allowing you to easily create signed upload URLs server-side to then upload assets from the client-side -- no matter which tech you choose to use for handling authentication for your app.
To facilitate this, we can create an API route in Next.js to generate these signed URLs:
The createSignedUploadUrl
method returns a token
which we can then use on the client-side to upload the file selected by React Dropzone:
Custom Next.js Image loader for Supabase Storage
Supabase Storage also conveniently integrates with the Next.js Image paradigm, by creating a custom loader:
Now we just need to register the custom loader in the next.config.js
file:
and we can start using the Next.js Image component by simply providing the file path within Supabase Storage:
_10<Image_10 alt="Expert image"_10 className="aspect-square rounded-md object-cover"_10 src="your-bucket-name/image.png"_10 height="64"_10 width="64"_10/>
Supabase Vercel Integration for one-click deploys
Supabase also provides a Vercel Integration which makes managing environment variables across branches and deploy previews a breeze. When you connect your Supabase project to your Vercel project, the integration will keep your environment variables in sync.
And when using the Vercel Deploy Button the integration will automatically create a new Supabase project for you, populate the environment variables, and even run the database migration and seed scripts, meaning you're up and running with a full end-to-end application in no time!
Contributing to open source
Both Cal.com and Supabase are on a mission to create open source software, therefore this new platform starter kit is of course also open-source, allowing you to spin up your own marketplace with convenient scheduling in minutes! Of course this also means that you are very welcome to contribute additional features to the starter kit! You can find the repository on GitHub!