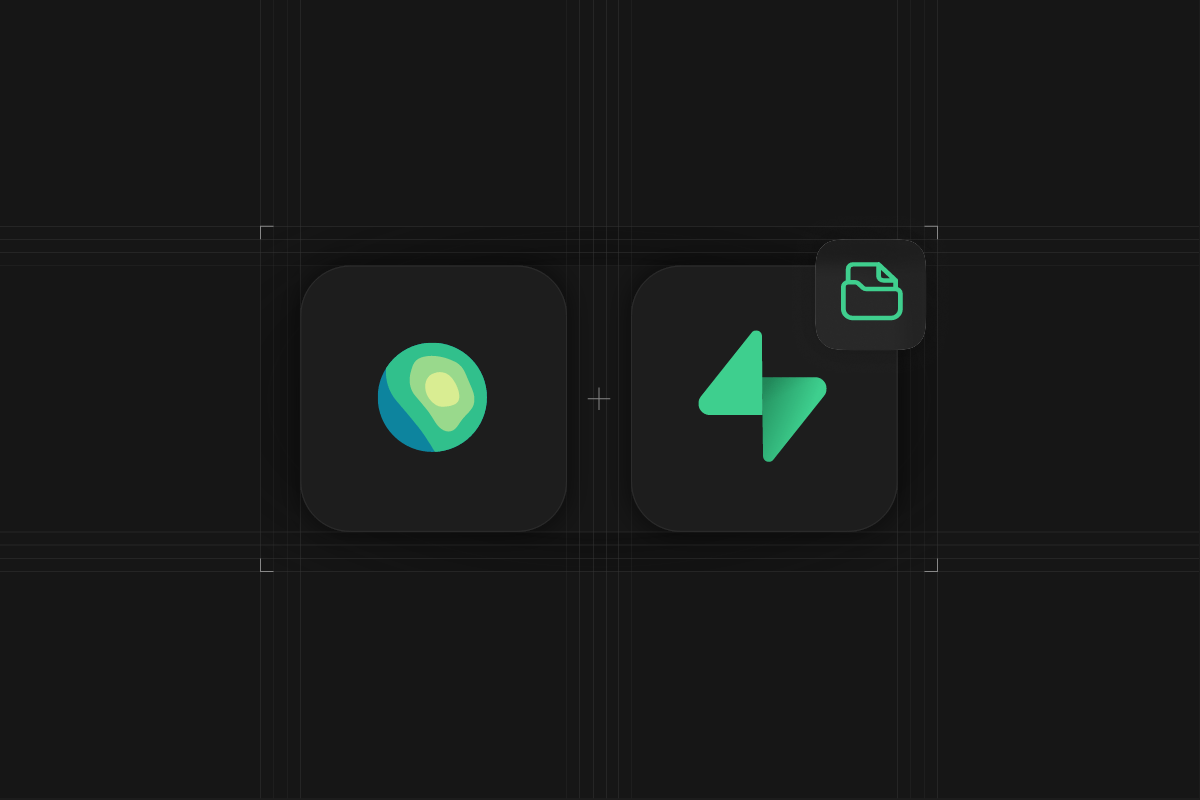
Protomaps is an open source map of the world, deployable as a single static file on Supabase Storage.
In this tutorial, you will learn to
- Use Protomaps to excract an area into a static PMTiles file.
- Upload the PMTiles file to Supabase Storage.
- Use MapLibre to render the Map onto a Web Page.
- Use Supabase Edge Functions to restrict File Access.
Extract an area into a static PMTiles file
Protomaps provides a pmtiles
CLI that can be used to cut out certain areas from the world map and compress those into a single static file.
For example, we can extract a small area around Utrecht in the Netherlands like this:
_10pmtiles extract https://build.protomaps.com/20240618.pmtiles my_area.pmtiles --bbox=5.068050,52.112086,5.158424,52.064140
Note: make sure to update the date to the latest daily build!
This will create a my_area.pmtiles
file which you can upload to Supabase Storage.
Upload the PMTiles file to Supabase Storage
In your Supabase Dashboard navigate to Storage
and click "New Bucket" and create a new public bucket called public-maps
.
Upload the my_area.pmtiles
file created earlier to your public bucket. Once uploaded, click the file and tap "Get URL".
Supabase Storage supports the required HTTP Range Requests out of the box, allowing you to use the public storage URL directly from your maps client.
Use MapLibre to render the Map
PMTiles easily works with both MapLibre GL and Leaflet. In our example we wil use MapLibre GL, which is a TypeScript library that uses WebGL to render interactive maps from vector tiles in a browser.
This is a vanilla JS example which uses CDN releases of the libraries. You can very easily adapt it to work with React as well, for example using the react-map-gl library.
Use Supabase Edge Functions to restrict Access
A public Supabase Storage bucket allows access from any origin, which might not be ideal for your use case. At the time of writing, you're not able to modify the CORS settings for Supabase Storage buckets, however you can utilize Supabase Edge Functions to restrict access to your PMTiles files, allowing you to even pair it with Supabase Auth to restrict access to certain users for example.
In your Supabase Dashboard, create a new private storage bucket called maps-private
and upload your my_area.pmtiles
file there. Files in private buckets can only be accessed through either a short-lived signed URL, or by passing the secret service role key as an authorization header. Since our Edge Function is a secure server-side environment, we can utilize the latter approach here.
Using the Supabase CLI, create a new Edge Function by running supabase functions new maps-private
, then add the following code to your newly created function:
If you want to further restrict access based on authenticated users, you can pair your Edge Function with Supabase Auth as shown in this example.
Lastly, we need to deploy our Edge Function to Supabase by running supabase functions deploy maps-private --no-verify-jwt
. Note that the --no-verify-jwt
flag is required if you want to allow public access from your website without any Supabase Auth User.
Now we can simply replace the public storage URL with our Edge Functions URL to proxy the range requests to our private bucket:
Now go ahead and serve your index.html
file, for example via Python SimpleHTTPServer: python3 -m http.server
and admire your beautiful map on localhost:8000!
Expo React Native
As you might know, I'm a big React Native fan, and when writing this tutorial I was very excited about making this work in Expo mobile apps also.
Unfortunately, at the time of writing, custom protocols are not supported in maplibre-react-native. There is an issues tracking this here, so if there are any native mobile wizards out there, I'd very much appreciate your contributions!
In the meantime, however, the Expo team had a great idea, what about leveraging React DOM components, which are currently experimentally supported in Expo SDK 52 preview.
This approach allows you to utilize react-map-gl and maplibre-gl-js across your Expo web and mobile apps.
Do you prefer the audio-visual learning? Watch the video guide!.
Or jump straight into the code.
Follow the steps to install the canary release.
To render a React component to the DOM, add the 'use dom' directive to the top of the web component file:
Inside the native component file, import the web component to use it:
Conclusion
Protomaps is a fantastic open source project that allows you to host your own Google Maps alternative on Supabase Storage. You can further extend this with powerful PostGIS capabilities to programmatically generate Vector Tiles which we will explore in the next post in this series. So make sure you subscribe to our Twitter and YouTube channels to not miss out! See you then!