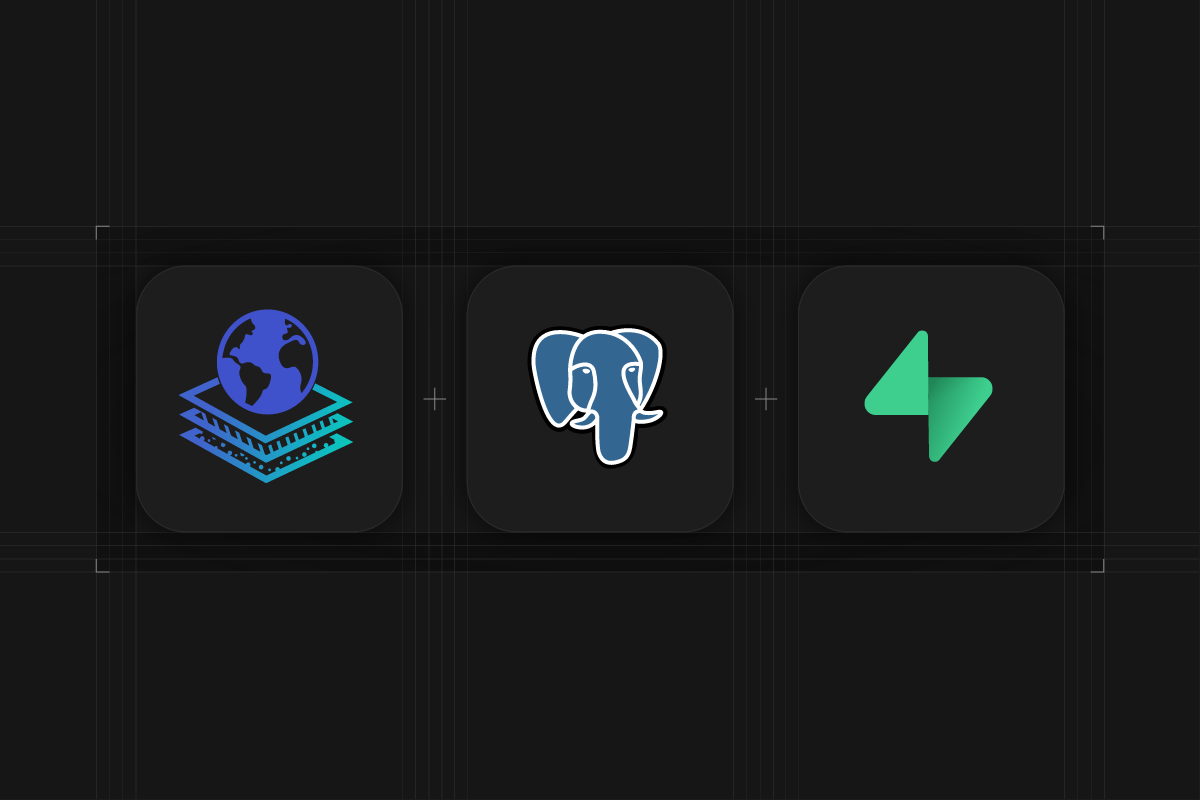
Overture Maps Foundation is a Joint Development Foundation Project initiated by Amazon, Meta, Microsoft, and tomtom, aiming to create reliable, easy-to-use, and interoperable open map data.
Overture Maps allows us to download open map data, like places of interest, as GeoJSON which we can transform into SQL and ingest into our Postgres database on Supabase.
Using PostGIS we can then programmatically generate vector tiles and serve them to our MapLibre GL client using supabase-js.
Vector tiles are packets of geographic data, packaged into pre-defined roughly-square shaped "tiles" for transfer over the web. Map data is requested by a client as a set of "tiles" corresponding to square areas of land of a pre-defined size and location.
Especially for large datasets, this has the benefit that the data transfer is greatly reduced because only data within the current viewport, and at the current zoom level needs to be transferred.
In this tutorial, you will learn to
- Use Overture Maps to download open map places data in GeoJSON format.
- Use GDAL ogr2ogr to transform GeoJSON into SQL statements.
- Import location data and JSON metadata into your Supabase Postgres database using psql.
- Use PostGIS'
ST_AsMVT
to aggregate a set of rows corresponding to a tile layer into a binary vector tile representation. - Use MapLibre's
addProtocol
to visualize large PostGIS tables by making remote procedure calls with supabase-js. - Use supabase-js to fetch additional JSON metadata on demand
Download open map data with Overture Maps
Overture Maps provides a python command-line tool to download data within a region of interest and converts it to several common geospatial file formats.
We can download places in Singapore into a GeoJSON file with this command:
_10overturemaps download --bbox=103.570233,1.125077,104.115855,1.490957 -f geojson --type=place -o places.geojson
Depending on the size of the bounding box this can take quite some time!
Transform GeoJSON into SQL
In the next step, we can use GDAL ogr2ogr to transform the GeoJSON file into a PostGIS compatible SQL file.
You can install GDAL
via homebrew brew install gdal
or follow the download instructions.
_10PG_USE_COPY=true ogr2ogr -f pgdump places.sql places.geojson
Import location data into Supabase
Enable the PostGIS extension on your Supabase Database on a dedicated separate gis
schema. To do so you can navigate to the SQL Editor and run the following SQL, or you can enable the extension from the Database Extensions Settings.
As PostGIS can be quite compute heavy, we recommend enabling it on a dedicated separate schema, for example, named gis
!
_10CREATE SCHEMA IF NOT EXISTS "gis";_10CREATE EXTENSION IF NOT EXISTS "postgis" WITH SCHEMA "gis";
Import the open map data into a places
table in Supabase:
_10psql -h aws-0-us-west-1.pooler.supabase.com -p 5432 -d postgres -U postgres.project-ref < places.sql
You can find the credentials in the project connect page of your Supabase Dashboard.
Enable RLS and create a public read policy
We want the places data to be available publicly, so we can create a row level security policy that enables public read access.
In your Supabase Dashboard, navigate to the SQL Editor and run the following:
_10ALTER TABLE "public"."places" ENABLE ROW LEVEL SECURITY;_10_10CREATE POLICY "Enable read access for all users" ON "public"."places" FOR SELECT USING (true);
Generate vector tiles with PostGIS
To programmatically generate vector tiles on client-side request, we need to create a Postgres function that we can invoke via a remote procedure call. In your SQL Editor, run:
_44CREATE OR REPLACE FUNCTION mvt(z integer, x integer, y integer)_44RETURNS text_44LANGUAGE plpgsql_44AS $$_44DECLARE_44 mvt_output text;_44BEGIN_44 WITH_44 -- Define the bounds of the tile using the provided Z, X, Y coordinates_44 bounds AS (_44 SELECT ST_TileEnvelope(z, x, y) AS geom_44 ),_44 -- Transform the geometries from EPSG:4326 to EPSG:3857 and clip them to the tile bounds_44 mvtgeom AS (_44 SELECT_44 -- include the name and id only at zoom 13 to make low-zoom tiles smaller_44 CASE_44 WHEN z > 13 THEN id_44 ELSE NULL_44 END AS id,_44 CASE_44 WHEN z > 13 THEN names::json->>'primary'_44 ELSE NULL_44 END AS primary_name,_44 categories::json->>'main' as main_category,_44 ST_AsMVTGeom(_44 ST_Transform(wkb_geometry, 3857), -- Transform the geometry to Web Mercator_44 bounds.geom,_44 4096, -- The extent of the tile in pixels (commonly 256 or 4096)_44 0, -- Buffer around the tile in pixels_44 true -- Clip geometries to the tile extent_44 ) AS geom_44 FROM_44 places, bounds_44 WHERE_44 ST_Intersects(ST_Transform(wkb_geometry, 3857), bounds.geom)_44 )_44 -- Generate the MVT from the clipped geometries_44 SELECT INTO mvt_output encode(ST_AsMVT(mvtgeom, 'places', 4096, 'geom'),'base64')_44 FROM mvtgeom;_44_44 RETURN mvt_output;_44END;_44$$;
To limit the amount of data sent over the wire, we limit the amount of metadata to include in the vector tile. For example we add a condition for the zoom level, and only return the place name when the user has zoomed in beyond level 13.
Use supabase-js to fetch vector tiles from MapLibre GL client
You can find the full index.html
code on GitHub. Here we'll highlight how to add a new protocol to MapLibreGL to fetch the bas64 encoded binary vector tile data via supabase-js so that MapLibre GL can fetch and render the data as your users interact with the map:
With the supabase protocol registered, we can now add it to our MapLibre GL sources on top of a basemap like Protomaps for example:
On demand fetch additional JSON metadata
To limit the amount of data sent over the wire, we don't encode all the metadata in the vector tile itself, but rather set up an onclick handler to fetch the additional metadata on demand within the MapLibre GL popup:
Conclusion
PostGIS is incredibly powerful, allowing you to programmatically generate vector tiles from table rows stored in Postgres. Paired with Supabase's auto generated REST API and supabase-js client library you're able to build interactive geospatial applications with ease!
Want to learn more about Maps and PostGIS? Make sure to follow our Twitter and YouTube channels to not miss out! See you then!