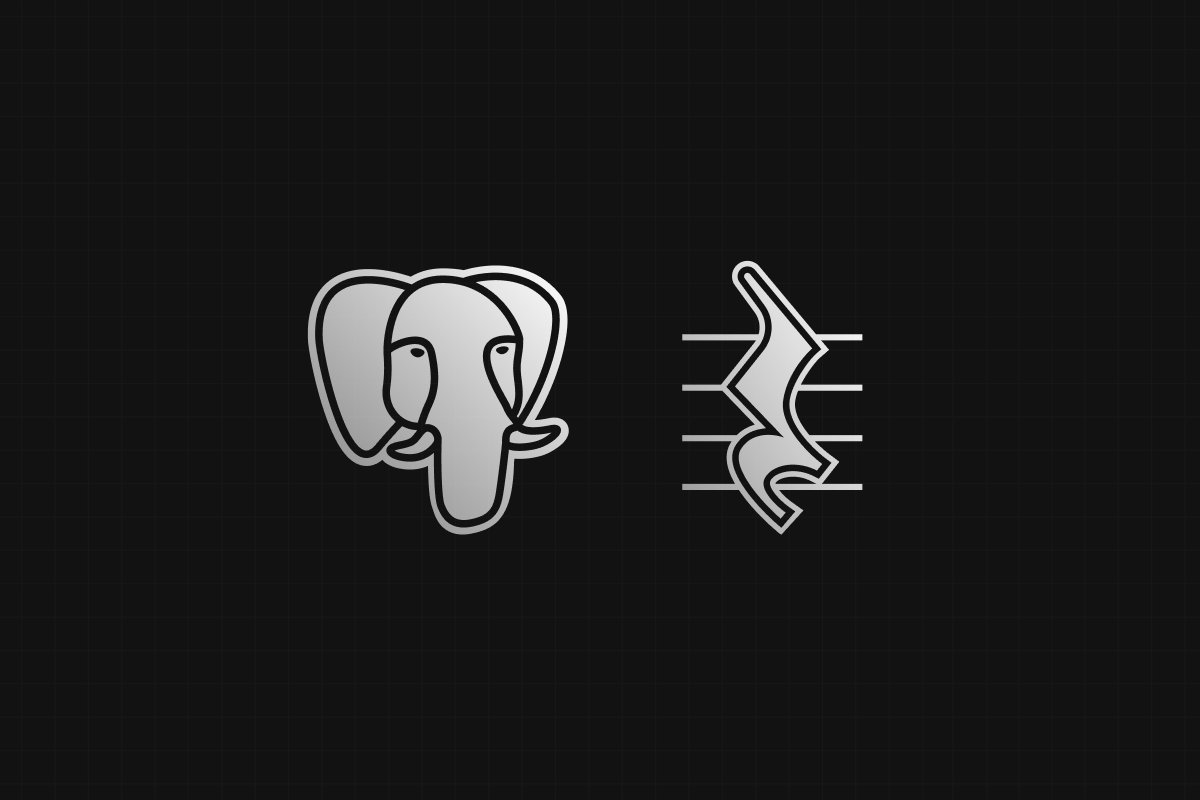
Behind every modern app is a sprawling backend: dozens of microservices, redundant APIs, managed databases, and gateways stitched together by developers. While this gives engineering teams control, it comes at a steep cost: time, maintenance overhead, and complexity that scales faster than your product.
For many teams, that complexity starts at the data layer. You model your database, write a set of REST endpoints, deploy them to servers, monitor them, patch them, and update them every time your schema changes. Repeat across environments, then across teams.
Supabase offers a different path. By exposing a secure, auto-generated REST and GraphQL API for every table, view, and stored procedure in the public schema in your Postgres database, Supabase compresses weeks of infrastructure work into minutes without sacrificing flexibility or control. This post explores how to use Supabase’s API layer.
How Supabase auto-generates APIs from your data model
Supabase exposes your Postgres database through a powerful RESTful interface, auto-generated by PostgREST. The moment you create a table or view, Supabase makes it accessible via a fully functional, queryable API with no boilerplate required. These endpoints are structured, predictable, and adhere to industry standards.
Let’s say you define a customers
table. Instantly, you get:
- GET
/customers
to fetch rows - POST
/customers
to insert - PATCH
/customers?id=eq.123
to update - DELETE
/customers?id=eq.123
to remove
And it doesn’t stop at basic CRUD. Supabase’s API layer supports a rich set of features out of the box:
- Filters and operators for advanced querying
- Pagination and ordering
- Embedded relationships with foreign key joins
- Exposing Postgres functions as RPC endpoints
- GraphQL support
- A dynamic connection pool that shrinks and grows based on traffic and whose max pool size grows as the instance size does
- Built-in observability with Prometheus metrics
Supabase automatically generates client libraries based on your schema. For example, here’s a JavaScript example using the official @supabase/supabase-js
client with auto-generated TypeScript types, querying an e-commerce-style schema with customers, orders, and products:
_15const { data, error } = await supabase_15 .from('orders')_15 .select(_15 `_15 id,_15 created_at,_15 total,_15 products (_15 id,_15 name,_15 price_15 )_15 `_15 )_15 .eq('customer_id', customerId)
Building custom API endpoints
Supabase provides two powerful options for building custom API endpoints when you need to go beyond standard CRUD operations: Database Functions and Edge Functions.
Postgres functions
Database Functions (also called stored procedures) allow you to encapsulate complex SQL logic inside the database itself. They are ideal for multi-step transactions, business rules, or performance-sensitive operations that work across multiple tables.
These functions can be exposed via the Supabase API using the .rpc()
call or accessed directly through the REST endpoint at /rpc/<function-name>
. Named parameters are passed as a simple JSON payload, making integration clean and declarative.
Here’s an example of a Database Function:
_10CREATE FUNCTION calculate_customer_discount(customer_id uuid) RETURNS numeric AS $$_10DECLARE_10 discount numeric;_10BEGIN_10 SELECT SUM(amount) * 0.1 INTO discount FROM orders WHERE customer_id = calculate_customer_discount.customer_id;_10 RETURN discount;_10END;_10$$ LANGUAGE plpgsql;
And you can call it from the client like this:
_10POST /rpc/calculate_customer_discount_10{_10 ”customer_id”: “uuid-of-customer”_10}
Here’s the TypeScript example of calling a Database Function using auto-generated types:
_10const { data, error } = await supabase.rpc('calculate_customer_discount', {_10 customer_id: 'uuid-of-customer',_10})
Supabase Edge Functions
Sometimes you need full flexibility outside the database, For example, you might want to integrate with external APIs or write business logic in TypeScript. For this, you’d want to use Supabase Edge Functions. These are custom serverless functions written in TypeScript and deployed globally at the edge, allowing you to define your own logic and expose it via HTTP.
Each function becomes its own endpoint:
_10https://<project-ref>.functions.supabase.co/<function-name>
For example, suppose you want to send a personalized discount email to a customer. You might create an Edge Function called send-discount
that could:
- Look up the customer by ID
- Apply business logic to determine eligibility
- Trigger an email via a third-party service
- Log the interaction
You would then call the Edge Function from your code like this:
_14const customerId = 'uuid-of-customer' // Replace with actual customer ID_14const projectRef = 'your-project-ref' // e.g. abcdefg.supabase.co_14const functionName = 'send-discount'_14_14const response = await fetch(`https://${projectRef}.functions.supabase.co/${functionName}`, {_14 method: 'POST',_14 headers: {_14 'Content-Type': 'application/json',_14 Authorization: `Bearer your-access-token`, // From Supabase Auth_14 },_14 body: JSON.stringify({_14 customer_id: customerId,_14 }),_14})
Edge Functions give you full flexibility to write this logic with access to Supabase Auth, your database, and any external APIs.
Use cases include:
- Creating custom checkout flows
- Handling webhooks (e.g. Stripe, OAuth)
- Validating orders before submission
- Integrating with AI tools or internal APIs
Edge Functions complement the auto-generated APIs, offering a path for deeper customization while avoiding the need to host your own backend services.
Rethinking architecture with less overhead
Supabase’s API layer eliminates the need to manually build and maintain middleware to serve data. Instead of managing a fleet of EC2 instances or containers just to provide an interface between your database and your client, Supabase is that interface.
This enables a radically simplified architecture:
- No more internal microservices per table or domain object
- No need to build an API gateway from scratch
- No separate deployment pipelines for frontend and backend developers
Everything is powered by your schema. Add a table, get an API. Change a column, the API reflects it. Need to restrict access? Just define a row-level security (RLS) policy.
For teams moving from hand-built APIs, this can reduce both technical debt and cloud spend. Customers routinely report that Supabase’s managed API layer simplifies onboarding for new developers and cuts build times. Quilia reduced development time by 75% with the Supabase Data API.
In practice, Supabase becomes a unified data plane: the single, secure interface for your application logic, internal services, and even external integrations.
Controlling access and ensuring security
Auto-generated does not mean exposed. When you use Postgres’s Row Level Security (RLS), Supabase’s APIs are secure by default. This means you write policies at the data layer, enforced by the database itself, not in brittle middleware code.
Want to restrict access to rows where user_id = auth.uid()?
One RLS policy handles it. Want public access to a products
table but private access to orders
? Define policies per table.
Supabase exposes the public schema by default to all users. It also allows mutations on the tables created there by default, unless RLS is specified. Developers should ensure that RLS is always used for security.
Authentication integrates seamlessly via Supabase Auth, which issues JWTs that are passed in every request. These tokens power identity-aware APIs and are validated by PostgREST natively.
Supabase also supports:
- API keys for service-to-service access
- Role-based permissions across environments
- Custom claims and token introspection
From a compliance perspective, Supabase offers regional project hosting (for example, in London or Frankfurt), dedicated infrastructure per project, and a shared responsibility model that supports GDPR-compliant deployments. Your data remains in your selected region, and Supabase provides Data Processing Agreements, Transfer Impact Assessments, and more.
Cost, speed, and maintenance tradeoffs
Custom API stacks are not just expensive in cloud bills. They are expensive in people hours. Every new endpoint adds scope. Every schema change becomes a deployment task. Every new hire needs to be onboarded into your bespoke architecture.
Supabase flips this equation. You no longer spend time writing endpoints that the platform can generate. You spend it building product.
In terms of cost:
- You reduce infrastructure: fewer compute nodes, no gateways, minimal DevOps
- You reduce time: instant APIs, schema-aligned contracts, no Swagger maintenance
- You reduce risk: fewer moving parts, fewer points of failure, consistent access control
For teams evaluating an architecture consisting of RDS and custom middleware versus Supabase, the total cost of ownership is usually lower with Supabase, though may in some cases converge. But the operational efficiency is not comparable. With Supabase, your backend just works without the constant maintenance burden.
Conclusion
Supabase’s API layer is not just a productivity boost. It is a backend reframe. By removing the need for hand-rolled REST and GraphQL endpoints, Supabase gives developers a secure, scalable, and schema-driven interface to their data.
It reduces infrastructure sprawl. It standardizes how you interact with your backend. And it lets your developers focus on the product, not the plumbing.
Whether you are replacing a fleet of microservices or spinning up a new prototype, Supabase’s auto-generated APIs let you move faster, with fewer errors, and more control.
Ready to try it yourself?